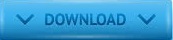
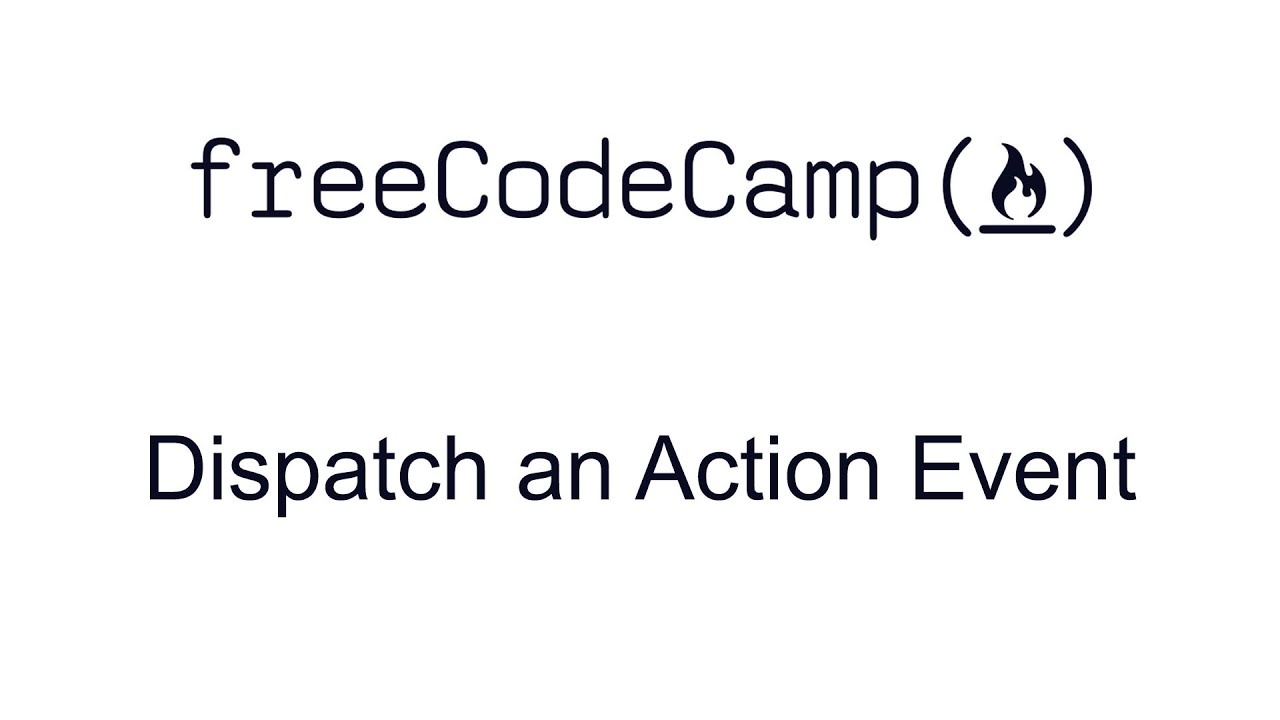

Now, every time I dispatch an action such as adding a todo, I can see it in the log.

Let's have a look at how our app runs with the wrapped dispatch function. Otherwise, I'm just going to leave the store as is. Finally, it's not a good idea to log everything in production, so I'll add a gate saying that if process and node is not production, then I'm going to run this code. Since the console group API is not available in all browsers, if it's not supported, I'll just return the raw dispatch as is. The action is blue, and the next state is green. Some browsers, such as Google Chrome, also offer an API to style console logs, and I'm going to add some colors to my logs. I want to log the next state as well, because after the dispatch is called, the state is guaranteed to be updated, so I can use store get state in order to receive the next state after the dispatch.
#REDUX DISPATCH CODE#
Now the calling code will not be able to distinguish between my function and the function provided by Redux, except that I also log some information. To preserve the contract of the dispatch function exactly, I am declaring the return value, and I'm calling the raw dispatch function with the action. Next, I will log the action itself so that I can see which action causes the change. I will log the previous state before dispatching the action by calling store get state. Some browsers like Chrome support using console group API to group several log statements under a single title, and I'm passing the action type in order to group several logs under the action type. It will return another function with the same signature as dispatch, which is a single action argument. It's going to wrap the dispatch provided by Redux, so it reads the raw dispatch from store.dispatch. I'm creating the new function called add login to dispatch, that accepts store as an argument. Now that my state shape is more complex, I want to override the store dispatch function to add some console logs there so that I can see how the state is affected by the actions. Middleware : ( getDefaultMiddleware ) => getDefaultMiddleware ( ). Import visibilityReducer from './visibility/visibilityReducer'Ĭonst debounceNotify = _. Import todosReducer from './todos/todosReducer' If it is an object of slice reducers, like from 'redux-batched-subscribe' If this is a single function, it will be directly used as the root reducer for the store. * If you only need to add middleware, you can use the `middleware` parameter instead.Įnhancers ? : StoreEnhancer | ConfigureEnhancersCallback * and should return a new array (such as ``). * function that will receive the original array (ie, ``), * If you need to customize the order of enhancers, supply a callback * All enhancers will be included before the DevTools Extension enhancer. * this must be an object with the same shape as the reducer map keys.
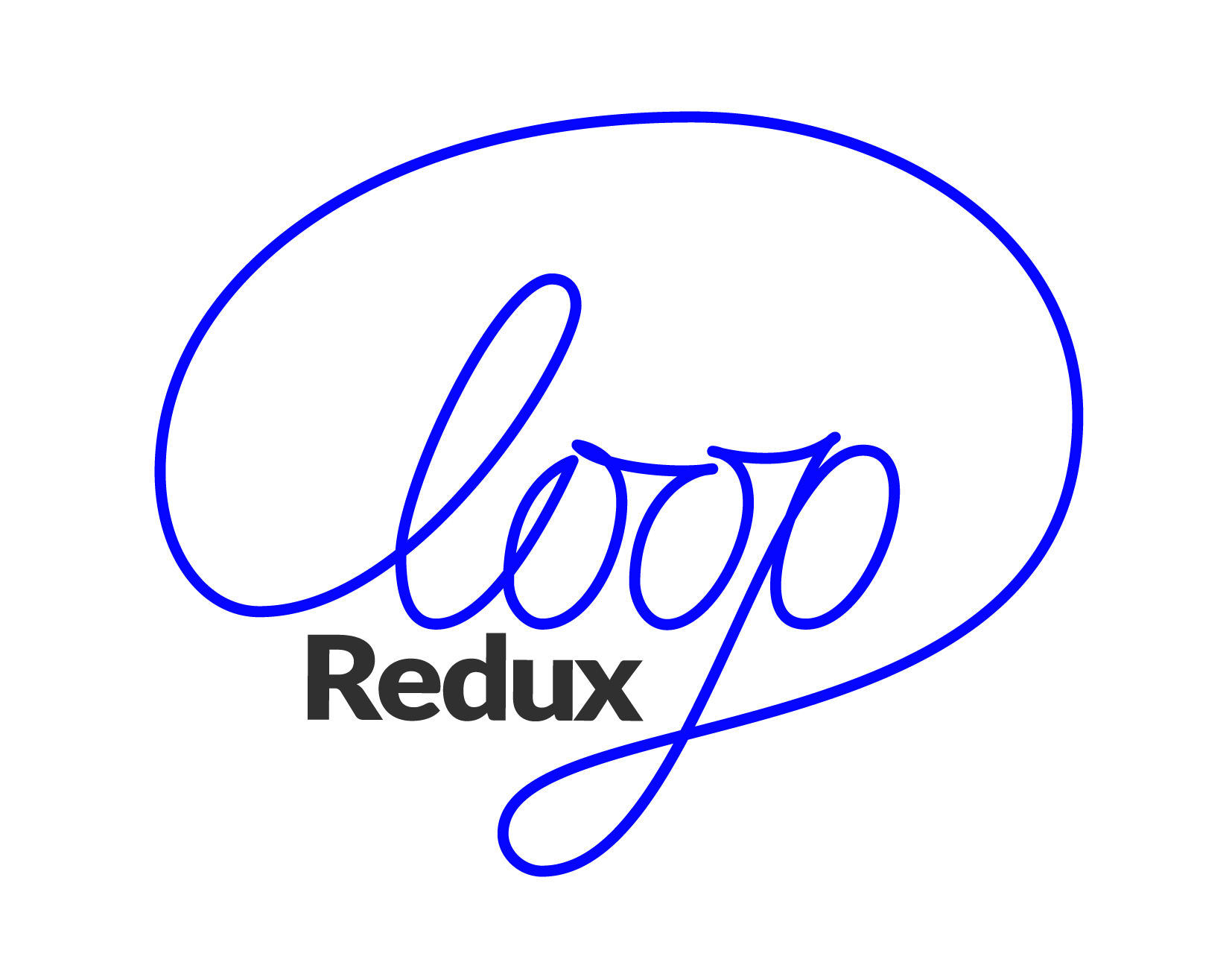
* function (either directly or indirectly by passing an object as `reducer`), If you use `combineReducers()` to produce the root reducer * from the server in universal apps, or to restore a previously serialized * You may optionally specify it to hydrate the state * The initial state, same as Redux's createStore. * Additional configuration can be done by passing Redux DevTools options * Whether to enable Redux DevTools integration. Middleware ? : ( ( getDefaultMiddleware : CurriedGetDefaultMiddleware ) => M ) | M * the set of middleware returned by `getDefaultMiddleware()`. * An array of Redux middleware to install. * object of slice reducers that will be passed to `combineReducers()`. * A single reducer function that will be used as the root reducer, or an Interface ConfigureStoreOptions = Middlewares
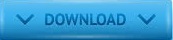